4. Scripts and styles
There are going to be occasions when you’ll want to remove JavaScript or CSS files that are loaded by the parent theme. You may also want to add your own JS or CSS files.
Just like before, you must first search the parent theme for the code that actually includes those files. The functions that you must look for are wp_enqueue_script() for JS scripts and wp_enqueue_style() for CSS styles. Here’s an example of something you might find in the parent theme:
/* PARENT THEME FUNCTIONS.PHP */ function add_scripts() { wp_enqueue_script( 'animation-js', get_template_directory_uri() . '/js/animated.js' ); } function add_styles() { wp_enqueue_style( 'custom-css', get_template_directory_uri() . '/css/custom.css' ); }
So we’re looking for wp_enqueue_*() functions.Β As you can see both functions use 2 parameters. The one that interests you right now is the first one. That’s the ‘handle’ or the name WordPress recognizes that script or style by. The second parameter is the location of the actual script / style file.
So all you have to do to remove the script or style from WordPress is add something like this to your child theme:
/* CHILD THEME FUNCTIONS.PHP */ function child_remove_scripts() { // using the deregister function only needs the 'handle' or name of the script wp_deregister_script('animation-js'); } // the function we created needs to be attached to the wp_enqueue_scripts hook add_action('wp_enqueue_scripts','child_remove_scripts'); /* SAME THING FOR STYLES */ function child_remove_styles() { wp_deregister_style('custom-css'); } add_action('wp_enqueue_styles','child_remove_styles');
And now that you’ve removed some probably useless scripts and styles you might want to add some of your own. Adding scripts and styles is even easier. You pretty much have to use the same code that you were searching for in the parent theme.
/* CHILD THEME FUNCTIONS.PHP */ function child_add_scripts() { wp_enqueue_script( 'child-animation-js', get_stylesheet_directory_uri() . '/js/animated.js' ); } add_action('wp_enqueue_scripts','child_add_scripts'); // Exactly the same thing with styles function child_add_styles() { wp_enqueue_style( 'child-css', get_stylesheet_directory_uri() . '/css/custom.css' ); } add_action('wp_enqueue_styles','child_add_styles')
As you can see you need to create a function in which you load your scripts or styles via the wp_enqueue_script or wp_enqueue_style functions. Then you load your newly created function via the wp_enqueue_scripts or wp_enqueue_styles hooks (notice the plural difference?).
The first parameter of the enqueue functions is easy. It’s just the handle – a name that you give it. The second parameter is the link to the file you’re adding. And as you can see it isn’t a traditional address like http://www.yoursite.com/wp-content/themes/mantra-child/css/custom.css. It uses the get_stylesheet_directory_uri() function instead. That prints an address up to your child theme’s directory (the directory that has the current style.css file inside) so you don’t have to remember WordPress’ file structure and, more importantly, the child theme stays valid even if you decide to switch domain names at a certain point in the future.
There’s another function get_template_directory_uri() that prints the link up to the parent theme’s directory. To make it all clear, using Mantra and our newly created mantra-child theme, here’s what each of the functions prints:
get_stylesheet_directory_uri() prints ‘http://www.yoursite/wp-content/themes/mantra-child/‘
get_template_directory_uri() prints ‘http://www.yoursite/wp-content/themes/mantra/‘
Using these two functions you can easily access all files both in your parent and your child theme.
5. Overriding theme files
Yet another way of altering the parent theme via a child theme is by overriding actual theme files. And that’s very easy to do: except for functions.php, most other files that you place in your child theme and have the same name as ones in your parent theme, override them and WordPress will only use the ones in your child theme. The usual ones are: header.php, footer.php, page.php, post.php, comment.php, category.php, content[-*].php.
For example let’s say you want to edit something inside header.php. Copy that file from your parent theme and place it in your child theme. You can now edit it here and get it to fit your every need. The parent theme will still have the original in case you get something wrong, and you are free to edit the one in your child theme in any way. This way you can easily edit important files like header.php, footer.php, page templates etc.
So you have things your way and you have a backup ready in case you screw something up. Plus you can freely update the parent theme without a worry. Now that’s what I call a win-win-win.
The same goes for the screenshot (screenshot.png file). Place a PNG image in your child theme’s folder to give your child theme some individuality and personality. You’ll see it on WordPress dashboard Β» Appearance Β» Themes together with the theme details (just like any other WordPress theme).
6. Firebug and beyond
Mainly for adding and editing CSS to your child theme you’ll need to see exactly what’s happening on the live site. You’ll need to see what class you want to hide, what border you want to make wider and what div you want to change the color of. To help you with this you have Firebug for Mozilla Firefox. There are similar tools for all other major browsers but if you want to get the best experience out of this there’s no replacement for Firebug.
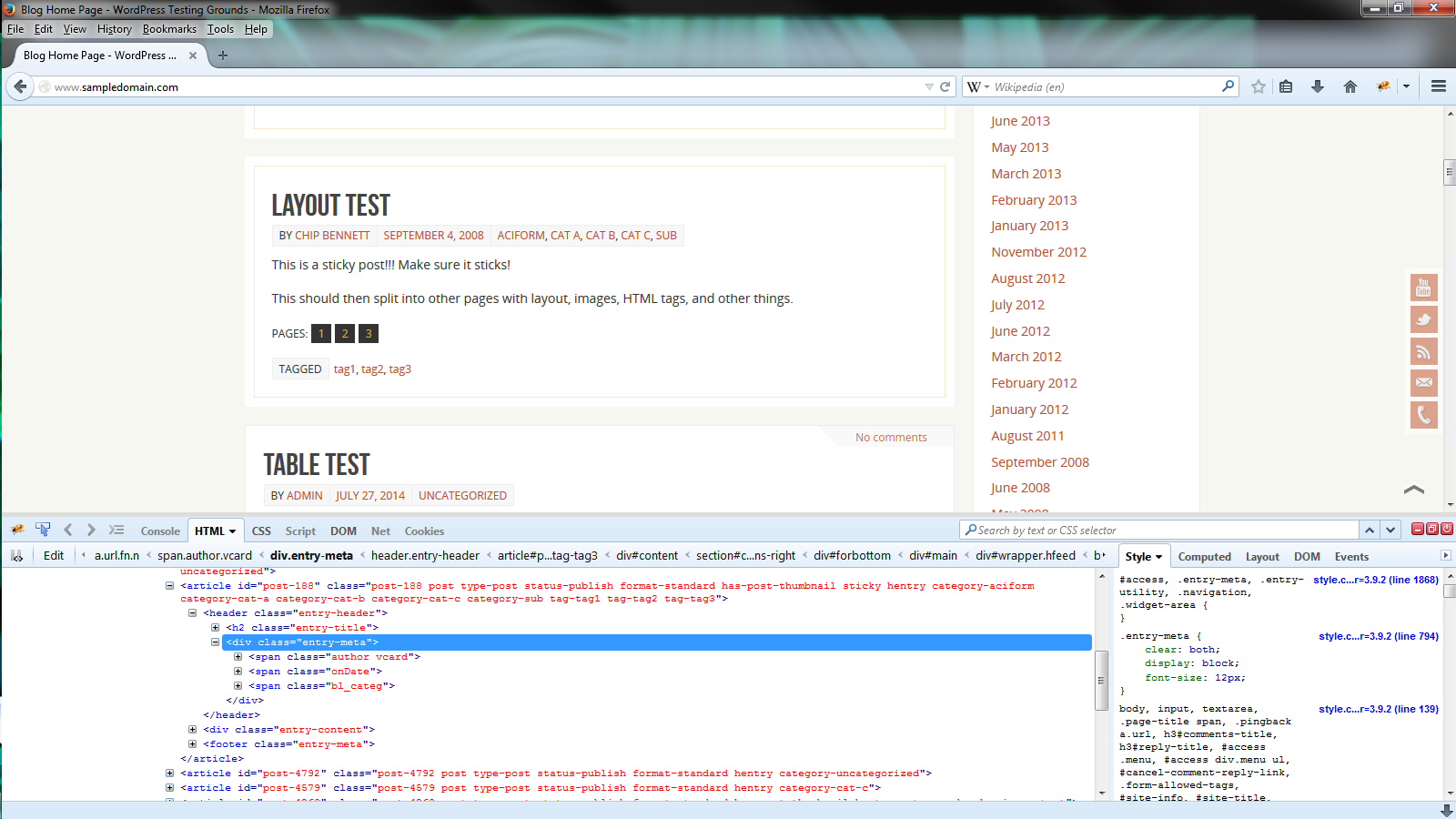
There’s a lot to cover about editing with Firebug so I’ll leave that for another tutorial and when that’s done you’ll see a link to it here.
Conclusion
Well that pretty much covers the basis of WordPress child themes. You’ll probably find out more on your own through experimentation and that’s actually the best thing about child themes. They give you the freedom to fly freely while providing an all-round safety net. Worst case scenario: you massacre style.css with copy-pasted CSS from some shady site and you type Hamlet inside functions.php. It’s a mess, your live site either looks like angry monkeys threw faeces at it or it doesn’t work at all and just throws some intelligible PHP errors at you. There’s nothing you can do and no one to help you. Then just delete the child theme folder via FTP and start over. You’ll learn even more this time, it’s a promise.
Thank you for helping people start in going deeper, I have several of the Cryout Themes and have been very happy with them. I’m having a little trouble.
I’ve added the folder and css file. I am able to activate the child them
When I go to my website I see the website but it is distorted, the Main menu seems to be distorted but the presentation page below this is OK.
This is the code for the style.css
Theme Name: My WordPress child theme
Theme URI: http://domain.com
Description: This is a child theme for the Parabola theme.
Author: Bob
Author URI: http://domain.com/me/
Template: parabola
Version: 1.0
*/
@import url(“../parabola/style.css”);
I suspect the problem is because I am using Parabola-nolink and it’s a “Child Theme”
so if you are using a “no-link” theme how does that affect things?
Should the name in the templete reflect that name and be:
Template: parabola-nolink
Thank you
I would add an image of how the wordpress install is distorted but don’t see an option to add images
Hello! If I’m using the Codex theme, which is already a child theme of Rosetta, do I still need to make a child theme of Codex? Or will my customizations within Codex remain even when an update is made to the Rosetta parent theme?
Here’s another question/anotherway of asking it…
Will there be regular updates made to the Codex theme itself that I’ll need to maintain? Or will there only be updates made to the Rosetta theme (so I’m safe)?
Thanks!
Codex is a child theme, you will be able to update its parent Roseta theme safely. However if we ever release an update for Codex itself that would overwrite any file changes you have applied to the child theme. Unfortunately there is no easy way around this as there is no direct support for grandchild themes in WordPress.
Would love to see this for Kahuna theme. Not to mimic the wordpress codex instructions, but each theme has special enqueues that don’t follow the codex pattern.
We’ll have to update the child themes tutorial (mostly the enqueues and style loading part) for newer standards and include actual child themes examples for all our themes. Hopefully we’ll find the time to do this soon.
Hi,
@import
It’s the old method, right?
Do not better put a code in the file functions.php, now?
thank you
Yes, using @import is a less efficient way. We’ll be updating the tutorial to suggest the currently recommended way (by using enqueues in the child theme).
Hi guys, I’m working on a Parabola Theme. I can’t able to create a Theme Child. I followed the same routing in the other website i buidl but in the back office I see just a corrupted theme… I can’t explain why. Can you help, please?
What does your child theme’s style.css file contain?
Hello,
Thank you for this great tutorial!
I am trying to make a child theme on Fluida basis. I need to replace the function fluida_setup() in /includes/setup.php which is hooked to ‘after_setup_theme’. Unfortunately the child function does not get called no matter where I hook it – I tried to init, after_setup_theme, setup_theme… Every time the parent function gets called.
Here is the code:
function fluida_setup_featured_imgs() {
// my custom code goes here
}
function child_remove_theme_actions() {
remove_action(‘after_setup_theme’,’fluida_setup’);
add_action(‘after_setup_theme’, ‘fluida_setup_featured_imgs’);
}
add_action(‘setup_theme’, ‘child_remove_theme_actions’);
Hello, I’m using Mantra theme and currently working for the child theme of it in order to keep my customization. I’ve been following this great tutorial for a while, but got stuck at “parent function removal’ thing and tried different ways in my whole day still haven’t figure it out. Hopefully you would like to help me out.
For instance, I was trying to modify the display of breadcrumbs, the related function is mantra_breadcrumbs(). So I have done this as follow what you written in tutorial:
function addon_mantra_breadcrumbs() {
// My customization code here
}
function replace_mantra_breadcrumbs() {
remove_action(‘cryout_before_content_hook’,’mantra_breadcrumbs’);
add_action (‘cryout_before_content_hook’,’addon_mantra_breadcrumbs’,0);
}
if($mantra_breadcrumbs==”Enable”) add_action (‘init’,’replace_mantra_breadcrumbs’);
I don’t know why the old function of breadcrumbs is still there…
function addon_mantra_breadcrumbs() {
// My customization code here
}
function addon_remove_mantra_functions() {
remove_action ('cryout_before_content_hook','mantra_breadcrumbs',0);
}
if($mantra_breadcrumbs=="Enable") {
add_action('init','addon_remove_mantra_functions');
add_action('cryout_before_content_hook','addon_mantra_breadcrumbs',0);
}
Well, that works somehow. thanks a lot!
Great article. I’ve been wanting to start building off of child themes and this really helped me understand it all.
Thanks! Now it is clear how to do Child Themes. I will try… ))
The attitude of this tutorial is really weird. I don’t think that serial killer joke is very funny, what if I actually did have a long term history of alcohol and drug abuse? It’s not really something to laugh about. I have a lot of friends with serious alcohol and drug problems and a history of abusive families, it doesn’t make them serial killers..it makes them people with difficult problems they are trying to get through. The whole mood of this tutorial is kind of aggressive. I know it’s trying to be funny, but to me the humour is randomly dark and kind of weird. It’s good to have a modern, edgy copy on your website, which breaks the monotony of reading stock standard copy and has a sense of humour…but when it comes to directly insulting the reader, you’re going to lose interest from them. I stopped reading halfway through and just decided to change to a different theme.
Would it be possible to have this page as a printer friendly page, without the side column which is not needed on a printout.
Is nirvana-theme able to handle a child-version? I use nirvana-theme and by doing like described in this tutorial, wordpress reports that the child-theme cannot be activated because the parent theme is missing. But both folders exist: βMakeβ & βMake-childβ and the style.css in the child-folder is like explained above. What can i do?
Thanks. Helped a lot to understand child themes. Keep posting π
Nice tutorial, thanks!
What about modifying a class like the one we can find in tempera/includes/widgets.php? It seems to need a different procedure. Any tips?
Thanks
That’s a rather difficult task. You can’t actually edit that class, but you can clone it and then unhook the original class’ functionality and hook your own into place.
Hi, sorry would you kindly clarify then:
If I make my changes in the Nirvana Settings and the Nirvan Custom CSS window…will I LOSE those changes if I update the Nirvana theme in the future???
If I want to use a font OTHER than Google fonts or the supplied Nirvana fonts, will I need a CHILD THEME?
and
Am I able to install other plugins, for example, a Portfolio plugin that I like?? Will I need to have a Child Theme for that?
I’ve used Child Themes many times with another theme company, where the Child Theme was INCLUDED with the core theme. I made CSS edits, etc. to the Child Theme, and occassonally, some php code (for Excerpts, for example).
I’ve never used a theme where I had to CREATE a Child Theme, so I am a bit unclear on what happens to the core Nirvana theme changes that I would make under Nirvana Settings.
thanks very much, great theme, and excellent Forum support!!
Thank you for the info. If all your edits are made in the theme options panels, and the only edits above that are custom css in the custom css section of the theme options panel, is a child theme necessary?
Hi Ryan,
If theme settings and custom CSS are enough to make your changes then you don’t need a child theme. Only if you need heavier changes like editing actual code, creating new functions etc, then you’d need a child theme.
This is excellent. I have been in need of a child theme, but was always a little apprehensive to start one.
Just started the tutorial, and got to the point where you recommend FileZilla. Not a bit fan of that program. It stores FTP credentials (site, user name, password) in a plain text file in the user’s folder. Easily accessible by anyone (or any hacker). Big security hole.
I’d recommend WinSCP. Fully encrypted access, including a master password that you can set up. FileZilla works as an FTP client, but the plain-text user credential file is a big no-no for this computer security dweeb.
If you want details on the security hole in FileZilla, I wrote about it two years ago here http://securitydawg.com/insecure-filezilla-ftp-program/ . I’ve stopped using it since then. They may have fixed it, but the developers didn’t think it was a problem two years ago, and weren’t going to fix it then.
FilezillaCrypt π
Is every Theme able to handle a child-version? Might be a silly question, but I bought the “make”-Theme from TheThemeFoundry and by doing like described in this tutorial, wordpress reports that the child-theme cannot be activated because the parent theme is missing. But both folders exist: “Make” & “Make-child” and the style.css in the child-folder is like explained above ….. hm??
We don’t usually answer questions that are not related to our own creations, but themes need to be written to support child theme advanced functionality override. Otherwise, all you’re able to change via child themes is CSS and the standard page/section templates.
Also, the parent theme name is case sensitive, so “make” and “Make” are two different parent themes.
Hello Zed!
I appreciated your comment very much. THX 4 your remark. I will ask TheThemeFoundry people.
This might sound like a stupid question, but what is the purpose of a child theme? Is it placed on a subdomain or what exactly?
I suggest reading http://codex.wordpress.org/Child_Themes
Thanks. Great introduction to child themes. Now I need to get my hands dirty…. π
Be careful though. Things can quickly get explosive if your hands are dirty π
Is Mantra compatible with the Hueman parent theme?
Is Mantra a child theme?
Hi,
Not at all, two different themes
Mantra is a theme.of Cryout Creations
Hueman is another theme, updated by Nicolas GUILLAUME
This is just what I have been needing to educate myself on, Thanks so much! I’ve been able to use theme options for most of the websites I’ve worked on but was really in need of a child theme for my current project and saw the Cryout updates on the Tempera theme options page – it’s like you knew I just needed this short tutorial to acquaint myself with child themes π
First time using the Tempera theme by the way and I love the adaptability – will definitely be using it again!